【Unity】一定範囲に入ると追ってくるスクリプト
目的
あるオブジェクトを中心にした一定範囲にPlayerが入ったときに、そのオブジェクトが追いかけてくるというスクリプトを作る。
環境
Unity 2020 1.1f1
Windows10
完成系
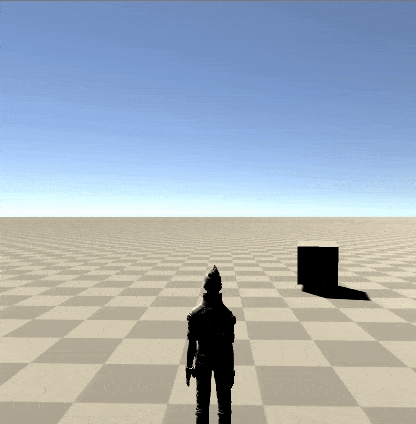
Step1 : プレイヤーの配置
Standard AssetsのEthanを使用します。配置の仕方はこちらで紹介しています。
配置したEthan。
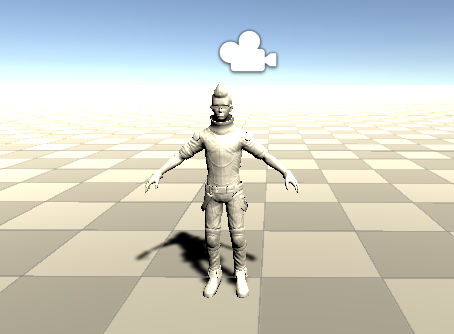
作成したEthanのTagをPlayerにしておきます。
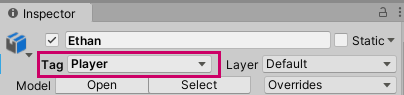
rigidbodyとcapusule colliderもつけておきます。
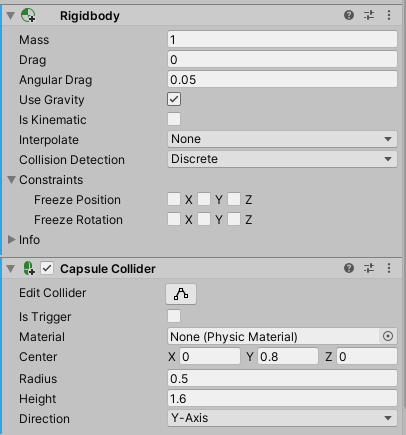
Step:2 追いかけてくるオブジェクトの配置
今回は敵の想定でCubeを使ってスクリプトを作成したいと思います。
まずはGameObject > 3D Object CubeからCubeを配置します。
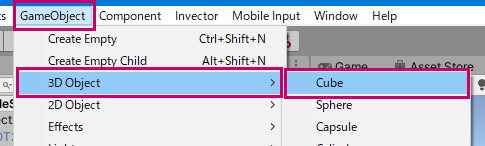
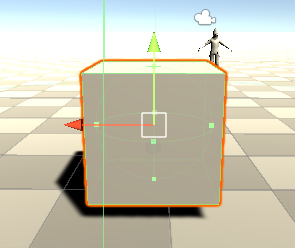
次に、CubeにCharacter ControllerとSphere Colliderを設定します。
Character ControllerはPlayerが一定範囲に入ったときにCubeを動かすために使用します。設定は特に触らずデフォルトのままにしておきます。
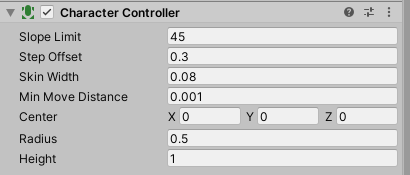
Shpere ColliderはPlayerが一定範囲に入ったかどうかを検出するために使用します。ここではIs Triggerにチェックを入れておきます。
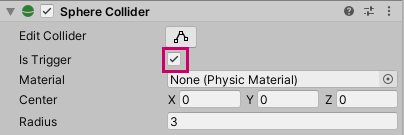
Step : 3 スクリプトの作成
次のようなスクリプトを作成し、Cubeへアタッチします。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Approach : MonoBehaviour
{
CharacterController Controller;
Transform Target;
GameObject Player;
[SerializeField]
float MoveSpeed = 2.0f;
int DetecDist = 8;
bool InArea = false;
// Use this for initialization
void Start()
{
// プレイヤータグの取得
Player = GameObject.FindWithTag("Player");
Target = Player.transform;
Controller = GetComponent<CharacterController>();
}
// Update is called once per frame
void Update()
{
if (InArea)
{
// プレイヤーのほうを向かせる
this.transform.LookAt(Target.transform);
// キューブとプレイヤー間の距離を計算
Vector3 direction = Target.position - this.transform.position;
direction = direction.normalized;
// プレイヤー方向の速度を作成
Vector3 velocity = direction * MoveSpeed;
// プレイヤーがジャンプしたときにキューブが浮かないようにy速度を0に固定しておく(空中も追従させたい場合は不要)
velocity.y = 0.0f;
// キューブを動かす
Controller.Move(velocity * Time.deltaTime);
}
//プレイヤーとキューブ間の距離を計算
Vector3 Apos = this.transform.position;
Vector3 Bpos = Target.transform.position;
float distance = Vector3.Distance(Apos, Bpos);
// 距離がDetecDistの設定値未満の場合は検知フラグをfalseにする。
if (distance > DetecDist)
{
InArea = false;
}
}
// プレイヤーが検知エリアにはいたら検知フラグをtrueにする。
private void OnTriggerEnter(Collider other)
{
InArea = true;
}
}
Step : 4 動作確認
あとはゲームスタートするだけです。これで最初のgifのような動きをするはずです。
以上
Unityを一から学ぶのにおすすめの本はコチラ