【Unity】Object starts to chase the player approaching
Overview
There is a sample the Cube Object starts to chase the player who goes in a certain area around it.
Environment
Unity 2020 1.1f1
Windows10
Picture of implementation
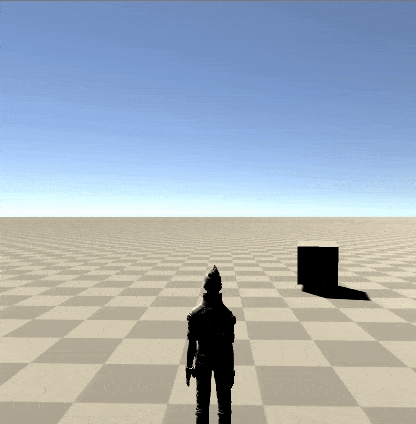
Step1 : Set the player
Ethan from the Standard Assets can be used as a human object. Flowwing article explains how to set Ethan and move him.
After place Ethan on Terrian, set the Tag in inspector “Player”.
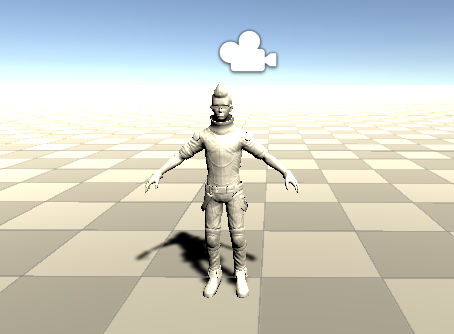
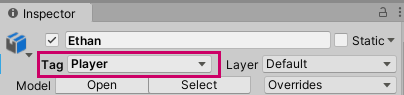
Adding rigidbody and capusule collider is needed.
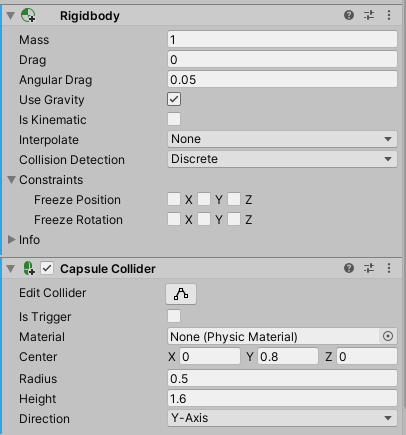
Step:2 Cube Object chasing player
Next, Set 3D Object Cube.
GameObject > 3D Object Cube
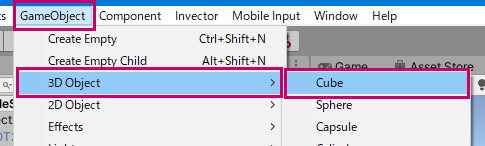
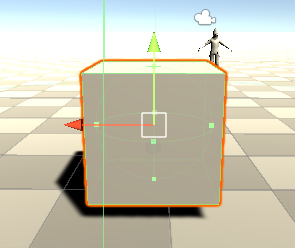
Adding Character Controller and Sphere Collider to the Cube Object.
Character Controller is useful to moving Cube. The parameters as Default is OK.
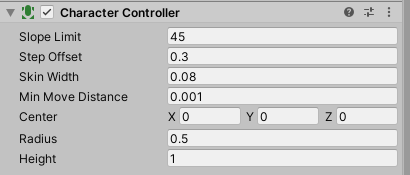
Shpere Collider can be used to detect Player is in certain area. Is Trigger should be checked.
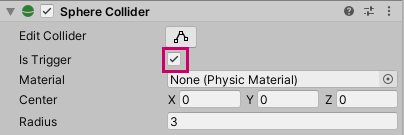
Step : 3 Script
Following script can realize Cube to chase player when he goes into cube area. Please attach this script.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | using System.Collections; using System.Collections.Generic; using UnityEngine; public class Approach : MonoBehaviour { CharacterController Controller; Transform Target; GameObject Player; [SerializeField] float MoveSpeed = 2.0f; int DetecDist = 8; bool InArea = false ; // Use this for initialization void Start() { // Get GameObject of Player by Tag Player = GameObject.FindWithTag( "Player" ); Target = Player.transform; Controller = GetComponent<CharacterController>(); } // Update is called once per frame void Update() { if (InArea) { // Look at Player this .transform.LookAt(Target.transform); // Getting direction from Cube object to Player Vector3 direction = Target.position - this .transform.position; direction = direction.normalized; // Velocity toward Player Vector3 velocity = direction * MoveSpeed; // Making y velocity 0 to avoid cube from floating toward y axis velocity.y = 0.0f; // Move cube Controller.Move(velocity * Time.deltaTime); } //Calculation of distance between the target object and this object Vector3 Apos = this .transform.position; Vector3 Bpos = Target.transform.position; float distance = Vector3.Distance(Apos, Bpos); // when distance between cube and player, cube stops tracing. if (distance > DetecDist) { InArea = false ; } } // Player gets inside area of cube. private void OnTriggerEnter(Collider other) { InArea = true ; } } |
Step : 4 Test
Please start game and check of them. You may see the pictures like gif animation at top of this page.